Universal Interaction Framework (UIF)
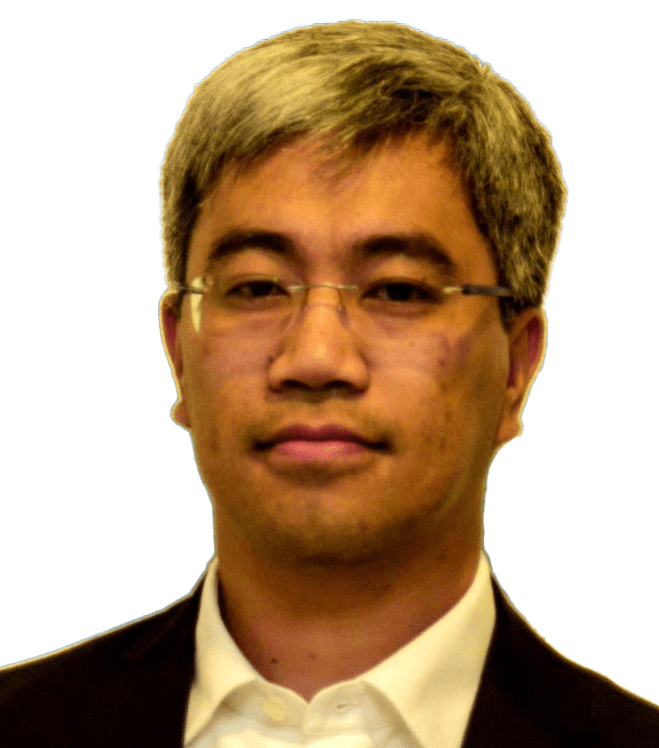
- Published on
Introduction
The Universal Interaction Framework (UIF) is the fifth foundational pillar of the Composable Frontend Architecture. Building on the first four pillars—Composable Execution Layer (CEL) for logic isolation, Dynamic Interface Mesh (DIM) for runtime composition, Adaptive Presentation Core (APC) for visual orchestration, and Event-Driven Component Network (ECN) for decoupled communication—UIF introduces a cohesive abstraction for handling diverse user interactions in a consistent, accessible, and platform-agnostic way. of the Composable Frontend Architecture. While CEL handles logic, DIM governs composition, APC adapts presentation, and ECN manages communication, UIF provides a universal interface abstraction layer that allows users to interact seamlessly across environments, input types, and device ecosystems.
UIF is not about styling or logic, but about building interaction primitives—events, gestures, focus flows, and accessibility patterns—that can be reused and adapted across platforms. UIF ensures that input handling and interaction design are decoupled from the rendering layer, and that apps behave predictably and inclusively no matter how users reach them.
UIF empowers developers to build accessible, multimodal, and cross-device experiences by exposing a consistent, context-aware interface layer on top of core interaction channels.
Why We Need UIF
As users engage with applications across devices—phones, tablets, laptops, smart TVs, kiosks, VR headsets—the variety of input mechanisms and interaction expectations grows. A single button might be clicked, tapped, keyboard-navigated, voiced, or gesture-activated depending on the device.
Traditionally, frontend developers handle these edge cases by layering specific event handlers (e.g., onClick
, onKeyDown
, onTouchStart
) onto components. But this quickly leads to:
- Redundant and tangled input logic.
- Fragile accessibility behavior.
- Unclear or inconsistent user expectations.
UIF was created to solve this.
UIF defines a layer of abstract interaction primitives—like "Activate", "Navigate", "FocusNext", or "Select"—that map to real-world user interactions depending on context. These primitives then emit standard events to connected components or systems like ECN or APC.
With UIF, developers can:
- Write interaction logic once and apply it across platforms.
- Decouple focus management and keyboard navigation from rendering.
- Support touch, keyboard, mouse, voice, and assistive tech with the same code.
- Design interaction-first, then render based on need.
Architecture Overview
To support advanced use cases such as eye tracking, progressive enhancement, and multimodal input orchestration, UIF integrates with other layers like ECN and CSEE. Below is the updated model.
[User Input Layer] ─┬─ (Keyboard)
├─ (Touch)
├─ (Mouse)
├─ (Voice)
└─ (Assistive Tech)
↓
+--------------------------+
| UIF Interaction Engine |
+--------------------------+
↓ ↓ ↓
[Focus Manager] [Gesture Mapper] [Command Dispatcher]
↓ ↓ ↓
[Component A] [Component B] [ECN Event Trigger]
Explanation:
- User Input Layer: All raw interaction channels feed into UIF.
- UIF Engine: Maps inputs into abstract semantic interactions.
- Focus Manager: Manages tab/focus order and ARIA navigation.
- Gesture Mapper: Handles swipes, taps, long presses.
- Command Dispatcher: Translates semantic actions into ECN events, component state changes, or visual feedback.
Historical Context and Prior Art
The challenges UIF addresses have deep roots in software history. As digital platforms evolved, so too did the complexity of user interaction. The inspiration for UIF comes from a wide range of systems and patterns that have long sought to abstract user input from the platform-specific implementation.
1. WAI-ARIA and Web Accessibility Standards
The Web Accessibility Initiative – Accessible Rich Internet Applications (WAI-ARIA) specification laid a foundation for UI behavior abstraction. It introduced roles, states, and properties to help assistive technologies interact with custom components.
What UIF Builds On:
- Abstract descriptions of behavior (e.g., 'button', 'menuitem', 'tab').
- Interaction patterns that work with screen readers and keyboard navigation.
- Emphasis on semantic behavior, not just visual styling.
2. Unity Input System & Game Engine Abstractions
In gaming, frameworks like Unity introduced input systems that unify gamepad, keyboard, and touch controls into a single abstraction.
What UIF Adopts:
- Platform-agnostic input mapping.
- Action-based models (e.g., "jump" instead of
keyCode 32
). - Multi-device support (handheld, console, PC).
3. Cross-Platform Design Systems (e.g., Fluent, Material Design)
Design systems like Google’s Material or Microsoft’s Fluent define not only components but interaction principles. They attempt to ensure consistency across web, mobile, and desktop by specifying motion, input zones, and feedback behavior.
What UIF Extends:
- These systems guide visual and tactile behavior but don't unify input handling. UIF provides the missing abstraction layer: how those interactions are triggered and how input is translated across devices.
4. Voice Interfaces and Conversational UI
Systems like Alexa Skills Kit, Google Assistant SDK, and SiriKit introduced intent-based user interaction. These voice-first interfaces are inherently abstract and rely on mapped intents instead of clicks or gestures.
How UIF Incorporates This:
- Treats voice input as another semantic signal.
- Maps spoken commands to actions like “Activate,” “FocusNext,” or “Dismiss.”
- Works seamlessly with visual, tactile, and auditory inputs.
5. Assistive Technology and HCI Research
Academic and industry research into Human-Computer Interaction (HCI) shaped a deeper understanding of how diverse users interact with digital systems—from keyboard-only users to people using eye tracking or adaptive switches.
UIF as a Unifier:
- Incorporates adaptive pathways.
- Reduces conditional UI logic by abstracting “what the user meant to do” from “how they did it.”
Implementation Examples
These examples demonstrate how UIF can unify multiple interaction sources under a single logic layer.
TypeScript: Define Semantic Actions
1 // uif/actions.ts
2 export type UIFAction = 'Activate' | 'FocusNext' | 'Dismiss';
3
4 export interface UIFContext {
5 inputType: 'keyboard' | 'mouse' | 'touch' | 'voice';
6 key?: string;
7 event: Event;
8 }
9 export function mapToUIFAction(ctx: UIFContext): UIFAction | null {
10 if (ctx.inputType === 'keyboard' && ctx.key === 'Enter') return 'Activate';
11 if (ctx.inputType === 'keyboard' && ctx.key === 'Tab') return 'FocusNext';
12 if (ctx.inputType === 'voice' && ctx.key === 'cancel') return 'Dismiss';
13 if (ctx.inputType === 'mouse' || ctx.inputType === 'touch') return 'Activate';
14 return null;
15 }
Explanation:
- Maps raw input context into semantic UIF actions.
- Enables platform-agnostic interaction logic.
React: Applying UIF to Components
1 import { mapToUIFAction } from './uif/actions';
2
3 export function UIButton({ onActivate }) {
4 const handleInteraction = (e) => {
5 const action = mapToUIFAction({
6 inputType: e.type === 'keydown' ? 'keyboard' : 'mouse',
7 key: e.key,
8 event: e
9 });
10 if (action === 'Activate') onActivate();
11 };
12 return (
13 <button onClick={handleInteraction} onKeyDown={handleInteraction}>
14 Submit
15 </button>
16 );
17 }
Explanation:
- Unified handler for both keyboard and click.
- Extensible to voice, gamepad, or accessibility tech with same interface.
Web Component: Focus and Dismiss Management
1 class ModalDialog extends HTMLElement {
2 connectedCallback() {
3 this.addEventListener('keydown', (e) => {
4 const ctx = { inputType: 'keyboard', key: e.key, event: e };
5 const action = mapToUIFAction(ctx);
6 if (action === 'Dismiss') this.close();
7 if (action === 'FocusNext') this.focusNext();
8 });
9 }
10 focusNext() {
11 const focusables = this.querySelectorAll('button, [tabindex]');
12 const current = document.activeElement;
13 const index = Array.from(focusables).indexOf(current);
14 const next = focusables[index + 1] || focusables[0];
15 next.focus();
16 }
17 close() {
18 this.setAttribute('hidden', 'true');
19 }
20 }
21 customElements.define('modal-dialog', ModalDialog);
Explanation:
- Shows how UIF action mapping simplifies keyboard navigation and modal behavior.
- Makes it easier to implement accessible focus flow with keyboard/voice parity.
Real-World Case Studies
UIF is rooted in challenges faced by large-scale applications needing consistent and accessible interaction models. The following case studies illustrate how some organizations embraced UIF-like patterns to streamline multimodal user experiences.
🏢 Microsoft – Fluent UI and Interaction Abstraction
Background: Microsoft's Fluent UI was designed to work across Windows, web, and mobile environments with consistent behavior.
Problem: Each platform (WinUI, React Native, Fabric) implemented its own gesture and focus handling, leading to duplicated work and inconsistent accessibility support.
UIF-Inspired Solution: Microsoft introduced shared abstractions for interaction behavior—such as focus rings, keyboard tabbing order, and pointer/touch parity—embedded into a centralized interaction layer consumed by each platform.
Results:
- Consistent interaction behavior across all devices.
- Easier implementation of accessibility features.
- Lower maintenance across platform-specific codebases.
🏢 Apple – Accessibility First Interactions in UIKit
Background: Apple’s UIKit and SwiftUI frameworks prioritize accessibility and multimodal input, supporting gestures, keyboards, switch control, and voice-over out of the box.
Problem: Third-party apps often implemented accessibility and interaction behavior manually, introducing regressions and poor user experience for non-mouse users.
UIF-Inspired Approach: UIKit components implement abstracted interaction behaviors such as UIAccessibilityAction
and UIFocusItem
. Apple developers write behavior once, and input handlers are automatically delegated to appropriate subsystems.
Results:
- Standardized interaction pathways for users of assistive technology.
- Reduced developer burden for complex input support.
- Broad coverage of real-world accessibility scenarios.
🏢 Figma – Unified Interaction Behavior Across Web & Native
Background: Figma is a design platform that must feel fluid on desktop browsers and hybrid mobile environments.
Problem: Initial prototypes handled keyboard shortcuts, pointer events, and multitouch gestures separately. UI interactions like selection, resizing, and nudging behaved differently depending on device.
UIF-Like Refactor: Figma engineers introduced a semantic interaction map that defines core actions (e.g., Select, Activate, Drag, Duplicate) independently from raw event types.
Results:
- Improved interaction fidelity.
- Easier testing of user actions.
- Predictable accessibility features.
Developer Experience Stories
"Before UIF, we had five different ways to handle a button press depending on device. Now it's just one semantic action we map to." — Senior Frontend Engineer, Global Retail App
"UIF let us plug accessibility and gamepad support into a kiosk interface without changing the components. That’s magical." — Interaction Designer, Healthcare UX Platform
"We used to bolt on keyboard nav at the end. With UIF, we start with interaction design and let rendering come second." — Web Accessibility Advocate
Benefits of UIF
Benefit | Description |
---|---|
Cross-Device Input | Unifies mouse, touch, keyboard, voice, and assistive tech input. |
Interaction Reusability | Define actions like 'Activate' once, use them anywhere. |
Accessibility-First | Interaction logic is compatible with ARIA and input semantics by design. |
Fewer Bugs | Avoids redundant event handling and tangled if/else chains. |
More Predictable UX | UI feels consistent across devices, improving trust and usability. |
Advanced Features and Extensions
Security Considerations
UIF must defend against spoofed, synthetic, or untrusted events. Real-world examples include attackers simulating synthetic click events to bypass CAPTCHA or confirmation modals, or voice-controlled devices inadvertently triggering sensitive actions from overheard commands (like the infamous Alexa purchases triggered by TV ads). Frontends must distinguish between genuine user-initiated input and programmatically generated ones, especially when handling critical UI actions or authorization workflows. that could lead to unintentional actions.
Best Practices:
- Always check
event.isTrusted
before triggering sensitive actions. - Avoid executing critical behavior from unvalidated voice commands or synthesized events.
- Use sandboxed input evaluators for untrusted device or gesture input.
Declarative Interaction Mapping
Instead of defining logic imperatively, UIF actions can also be declared using structured config files or schemas:
// uif-map.json
{
"Activate": ["Enter", "Tap", "Click"],
"Dismiss": ["Escape", "Voice:cancel"],
"FocusNext": ["Tab"]
}
This improves maintainability and supports UI builders, low-code systems, and adaptive design systems.
Fallback Layers & Progressive Enhancement
UIF supports layering interactions so that if a preferred input type fails or is unavailable, the system degrades gracefully to another method.
Example Pathways:
- Eye-tracking fallback → switch navigation → keyboard
- Voice → keyboard → touch → mouse
UIF ensures user flows are never blocked due to limited device capabilities or accessibility tools.
→ fallback to keyboard.
- If pointer device is absent → use switch navigation.
Fallbacks ensure that apps are always operable and inclusive.
Testing Strategies
To test UIF:
- Use
@testing-library/react
to simulate keyboard and pointer. - Simulate UIF actions by dispatching mapped events.
- Use tools like Cypress or Playwright for end-to-end interaction coverage.
- Instrument ECN events to trace action propagation.
Emerging Inputs & Future Patterns
UIF is built for forward compatibility:
- Eye Tracking: Detect gaze on focusable regions → auto-trigger "FocusNext".
- Biometric Sensors: Use facial gestures, squeeze, or blink as mapped triggers.
- Environmental Signals: Adjust behavior based on ambient light, orientation, or noise.
These inputs can be mapped semantically, just like any traditional input.
Integration with Analytics & ECN
UIF actions can emit ECN events and analytics logs:
if (action === 'Activate') {
ecnHub.emit('user::activated', { source: 'button::submit' });
analytics.track('UIFActivate', { target: 'submitButton' });
}
This enables behavior tracking, accessibility auditing, and usage heatmaps—all tied to semantic interaction layers.
UIF is designed to be extended with:
- Eye tracking for gaze-based interaction.
- Biometric sensors (e.g. squeeze, nod, facial gesture).
- Environmental context (e.g. light sensor triggers interaction hints).
Visual Diagram: UIF Interaction Model
+----------------------------+
| User Input |
| (touch, keyboard, voice) |
+-------------+--------------+
↓
+---------------------+
| UIF Interaction Map |
+---------------------+
↓ ↓ ↓
'Activate' 'FocusNext' 'Dismiss'
↓ ↓ ↓
+----------+ +-------------+ +------------+
| Button A | | Modal Dialog| | Snackbar UI|
+----------+ +-------------+ +------------+
Explanation:
- Input methods are abstracted into semantic interactions.
- UIF maps those to action handlers across multiple components.