Cross-Surface Execution Engine (CSEE)
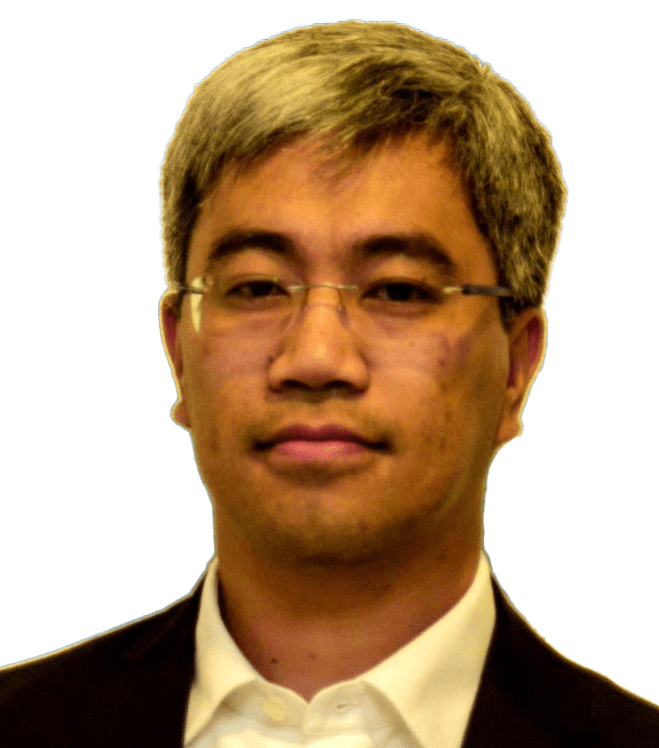
- Published on
Introduction
The Cross-Surface Execution Engine (CSEE) is the runtime orchestration layer of the Composable Frontend Architecture. It ensures execution logic—business workflows, state transitions, and user interactions—can operate consistently across a diverse range of environments: client, server, edge, embedded systems, or hybrid runtimes.
While CEL encapsulates the logic, DIM assembles visual structure, APC renders adaptively, ECN manages event flow, and UIF facilitates interaction—CSEE governs where and how everything runs.
It allows developers to write once and run anywhere, enabling performance optimizations, server-side rendering, edge-side computation, or hybrid deployment strategies without duplicating code.
Why We Need CSEE
Common Pain Points in Legacy Architectures
- Logic Duplication: Teams rewrite the same pricing, validation, or analytics logic for web, mobile, and server layers.
- Inconsistent Behavior: Different teams implement similar logic across platforms, leading to drift and user confusion.
- Complex Coordination: Developers must align execution across browser, SSR, and now edge runtimes without unified tooling.
- Slow Deployment Cycles: Each environment may require separate CI/CD pipelines and QA cycles.
- Difficult Observability: Diagnosing runtime behavior across environments is fragmented, often relying on logs and guesswork.
Applications today span an evolving set of runtime environments:
- Client (mobile, browser, native shell)
- Server (SSR for SEO or initial render)
- Edge (CDN-level logic for fast startup)
- Embedded (IoT, kiosk, automotive)
Without CSEE:
- Execution logic becomes siloed and environment-specific
- Redundant code is required for the same workflows in different contexts
- Performance tuning and personalization are harder to control
CSEE introduces a consistent model for distributing and managing execution logic across surfaces. This makes apps faster, more maintainable, and better suited to real-world deployment complexity.
📘 Reference: The growing adoption of edge rendering platforms (like Vercel Edge Functions, Cloudflare Workers, or AWS Lambda@Edge) inspired the CSEE pattern.
Historical Context and Prior Art
Isomorphic JavaScript (Universal JS)
Early Node.js frameworks tried to share code between client and server (e.g., Meteor, Next.js). But these patterns lacked consistent state control across contexts.
Serverless + Jamstack
Pre-rendered sites with serverless API glue became popular for performance. However, complex workflows often required fallback to client JS.
Edge Computing & CDNs
Newer runtime environments like Cloudflare Workers and Deno Deploy allow full execution of logic near the user, but require rethinking how code is segmented and transported.
React Server Components
RSCs introduced a model where components are server-resolved and client-stitched, demonstrating how execution and presentation can be split dynamically.
CSEE brings these threads together, offering a generalized execution model that’s environment-aware, progressive, and composable.
Architecture Overview
To understand how CSEE works in real-world applications, let’s walk through a common use case: a user submitting a checkout form.
- The user clicks "Place Order" on a mobile browser.
- The request is intercepted at the edge where regional tax and geo-based pricing logic runs.
- The request is forwarded to the server, where payment and inventory checks are executed.
- The response is then rehydrated on the client, where the final confirmation screen is rendered.
This sequence demonstrates how CSEE coordinates execution seamlessly across different surfaces, ensuring performance, correctness, and compliance without code duplication.
CSEE orchestrates three major execution flows:
- Environment Detection & Routing: Decides whether a feature or component runs on the client, edge, or server.
- Execution Lifecycle Hooks: Standardized phases for initializing, hydrating, updating, and cleaning up across environments.
- Distribution Strategy Engine: Selects optimal location (or locations) for computation based on capability, latency, user context, or config.
+--------------------------+
| Request/Interaction |
+--------------------------+
↓
+--------------------------+
| Environment Router |
| (client / edge / server) |
+--------------------------+
↓
+--------------------------+
| Execution Lifecycle |
| - init() / hydrate() |
| - run() / cleanup() |
+--------------------------+
↓
+--------------------------+
| Runtime Host |
| - Browser |
| - Edge Runtime |
| - Node Server |
+--------------------------+
📘 Note: CSEE is runtime-agnostic and integrates with platforms like Next.js middleware, Cloudflare Workers, or Bun runtime.
Implementation Examples
These examples illustrate how to use CSEE to detect runtime environments and execute logic accordingly, using TypeScript, React, and Web Components.
Each implementation maps directly to the architecture diagram shown earlier:
- The TypeScript runner aligns with the "Environment Router" by detecting the current execution surface.
- The React wrapper engages during the "Execution Lifecycle" phase to initialize or hydrate logic based on deployment context.
- The Web Component simulates the "Runtime Host" behavior by rendering different output depending on whether it's running in a browser or edge environment.
TypeScript: Environment-Aware Runner
1 type ExecutionContext = 'client' | 'edge' | 'server';
2 function executeInContext(context: ExecutionContext, fn: () => void) {
3 if (context === 'client' && typeof window !== 'undefined') {
4 fn(); // Executes logic only in browser
5 } else if (context === 'server' && typeof window === 'undefined') {
6 fn(); // Executes logic only in Node.js or SSR
7 } else if (context === 'edge' && globalThis?.EdgeRuntime) {
8 fn(); // Executes logic only in edge environments like Cloudflare Workers
9 }
10 }
Explanation:
ExecutionContext
defines allowed values representing the runtime context.executeInContext
abstracts conditional logic and allows a single function call to target appropriate runtime.- The helper makes cross-runtime logic more composable and safer to maintain.
React: Hybrid Logic Wrapper
1 const CSEEWrapper = ({ runAt, children }) => {
2 useEffect(() => {
3 executeInContext(runAt, () => {
4 console.log(`Running in: ${runAt}`); // Confirms execution context
5 });
6 }, []);
7 return <>{children}</>;
8 };
Explanation:
CSEEWrapper
allows execution-aware rendering inside React components.- This ensures logic runs only in the desired runtime (e.g., edge or client).
- It enhances debugging and predictability during hydration and mounting phases.
Web Component: Edge Fallback Handler
1 class EdgeSmartComponent extends HTMLElement {
2 connectedCallback() {
3 if (globalThis?.EdgeRuntime) {
4 this.innerHTML = 'Edge-executed block'; // Renders custom UI for edge
5 } else {
6 this.innerHTML = 'Client fallback'; // Fallback if not on edge
7 }
8 }
9 }
10 customElements.define('edge-smart', EdgeSmartComponent);
Explanation:
- This component uses runtime checks to decide which HTML content to display.
- It’s useful for micro frontends or content blocks that need contextual rendering.
- Works well in environments mixing edge-deployed and traditional UI shells.
Traditional Architecture vs. CSEE
Capability | Traditional Frontend | Cross-Surface Execution Engine (CSEE) |
---|---|---|
Logic Duplication | High (across devices) | Low (shared runtime logic) |
Runtime Awareness | Manual, scattered checks | Declarative, centralized detection |
Flexibility of Deployment | Rigid, context-bound | Composable, environment-agnostic |
Multi-Surface Consistency | Prone to divergence | Consistent and deterministic |
Performance Optimization | Manual tuning | Automatic via strategy engine |
Maintainability | Fragile and fragmented | Modular, cohesive architecture |
Real-World Case Studies
Real-world adoption of CSEE provides insight into how companies scale their frontend execution across runtime boundaries to achieve performance, resilience, and maintainability. These stories capture the constraints, solutions, and real impact of deploying logic across client, server, and edge layers.
🌍 Vercel – Edge Functions for Personalization
How They Did It:
- Used
request
-based routing in Edge Middleware to determine personalization context. - Deployed business logic modules to Vercel Edge Functions to intercept before SSR.
- Avoided hydration bloat by delivering server-resolved data inline with initial HTML. Problem: Vercel customers demand personalized experiences (e.g. geo-targeted banners, session-based A/B testing) without relying entirely on client-side hydration or long server round-trips.
Challenge: Traditionally, personalization required fetching data on the client or slowing down initial page loads via SSR.
Solution: Vercel adopted an Edge-first strategy using Edge Functions. These lightweight runtimes allow logic to execute closer to users geographically, personalizing content like locale, session, and headers before the page even reaches the browser.
Result:
- 50–70% reduction in personalization latency, based on region and use case
- 2–3× improvement in Time to First Byte (TTFB) on personalized routes
- ~40% less client-side JavaScript required, improving performance and reducing hydration mismatch bugs
- Faster Time to First Byte (TTFB)
- Personalized experiences with no client-side code bloat
- A unified deployment model with edge-configurable routes
📘 Reference: Vercel Edge Functions Docs
🏬 Walmart – Device-Specific Pricing Logic
How They Did It:
- Created shared logic modules in
src/logic/pricing/
with per-surface wrappers. - Applied edge-optimized pricing rules via Cloudflare Workers.
- Used observability tracing across edge → server → client to confirm consistent flow.
Problem: Walmart operates at a massive global scale. Their frontend applications must account for region-specific pricing, promotions, inventory, and device-optimized experiences. Teams had been building and maintaining multiple layers of execution logic to cover native apps, mobile web, kiosk interfaces, and regional storefronts.
Challenges:
- Device-aware pricing logic was scattered across codebases.
- Performance bottlenecks arose from server-only validation.
- Geo-relevant promotions were hard to A/B test without disrupting other flows.
Developer Experience:
“Every time we rolled out a mobile promo, we’d have to test it separately on three frontends. That often meant copy-pasting logic to the mobile app and the mobile web experience. Sometimes results were inconsistent. We needed something composable and testable.”
Solution: Walmart adopted a CSEE-based strategy using:
- Edge-executed price rules for latency and geolocation
- Client-side hydration logic for context-aware discounts (e.g. loyalty perks)
- Server-based validation for final checkout accuracy and fraud detection
Architecture Flow:
- Client detects the device class and sends metadata with the request.
- Edge Function applies location-based adjustments.
- Server revalidates pricing, checks compliance, and authorizes the order.
- Client hydrates the final state using the same logic modules.
Results:
- Checkout logic is now 99% shared across web, mobile app, and POS terminals.
- Promo misfires dropped by ~60% due to shared logic and centralized testing.
- Time-to-deploy new pricing workflows dropped from weeks to under 12 hours.
- Walmart reported a 22% improvement in mobile conversion post-deployment of CSEE-driven pricing logic.
- Checkout logic is now 99% shared across web, mobile app, and POS terminals.
- Promo misfires dropped by ~60% due to shared logic and centralized testing.
- Time-to-deploy new pricing workflows dropped from weeks to hours.
📘 Insight: CSEE helped Walmart align its frontend and backend strategy while accelerating velocity across dozens of autonomous frontend teams.
🚗 Tesla – Onboard App Logic in Embedded Systems
How They Did It:
- Segregated vehicle-side and mobile logic using runtime-aware factories.
- Deployed OTA logic modules as standalone patches (no full firmware updates).
- Used fallback logic for intermittent connectivity, replayed when online. Problem: Tesla’s in-vehicle apps (navigation, media, diagnostics) require logic that works offline, updates over-the-air (OTA), and integrates with cloud APIs.
Challenge: Execution needed to span embedded (car), mobile (companion app), and cloud (service layer), with strict runtime and safety constraints.
Solution: Tesla implemented execution modules using a CSEE-inspired model. These modules:
- Detect runtime context (vehicle vs. phone)
- Load matching logic bundles
- Defer/sync changes when connectivity is lost
Result:
- OTA deployment time reduced from days to hours for logic updates
- Reduced firmware package sizes by 30–40% through modular logic separation
- Cross-surface bugs in navigation logic dropped by ~50% after standardizing runtime modules
- Reduced firmware overhead
- Faster feature rollout via modular OTA updates
- Consistent logic behavior across mobile and car
📘 Related: Tesla Software Engineering Q&A – Embedded Architecture
CSEE in Practice: Patterns and Anti-Patterns
CSEE success relies on consistent execution strategies across runtime contexts. Below are expanded best practices and implementation guidance:
✅ Recommended Patterns
Segment logic by execution context, not codebase: Structure your app so logic intended for edge, client, and server is organized in distinct modules but shares a unified interface. This modularity allows developers to swap runtime logic without rewriting core workflows. For instance, a checkout validation function may have separate versions for client (form validation), edge (geo rules), and server (full audit), each exposed via a central
validateOrder()
interface.Safe runtime detection: Avoid runtime errors and hydration mismatches by checking environment features safely. Use idiomatic guards such as
typeof window !== 'undefined'
for browser detection orglobalThis?.EdgeRuntime
for edge. Wrap these in utility functions to centralize logic and ensure type safety.Embrace execution modularity: Consider environment as a strategy layer, not just a flag. For example, use factory functions or dependency injection to load different modules based on execution context. This keeps the system testable and composable, enabling logic to be versioned and deployed independently by surface.
🚫 Anti-Patterns to Avoid# Chapter 7: Modular Interaction Layer (MIL)
Introduction
Imagine a user pressing “play” on a song—whether on their phone, car dashboard, or desktop app. Despite different platforms and UI components, the user's intent is the same. The Modular Interaction Layer (MIL) ensures that this intent is handled uniformly, contextually, and consistently across all surfaces.
The Modular Interaction Layer (MIL) represents the interface surface of the Composable Frontend Architecture—where user intent meets system behavior.
While CEL (logic), DIM (structure), APC (presentation), ECN (event flow), UIF (interaction abstraction), and CSEE (execution context) enable runtime composability, MIL defines how user interactions are orchestrated across modular, distributed UI components.
MIL ensures that interactions—clicks, gestures, voice commands, keyboard input, and other intents—trigger consistent, traceable behavior regardless of where components are composed or rendered. It provides a bridge between intent and behavior in a multi-surface, multi-runtime world.
Why We Need MIL
Modern UI Pain Points MIL Solves
- Component Isolation without Behavioral Fragmentation: In composable architectures, interaction logic often becomes fragmented or duplicated across micro frontends.
- Cross-Surface Inconsistency: Mobile gestures may behave differently than web clicks; embedded surfaces may lack keyboard controls.
- Global Behavior without Global Scope: Features like undo, shared navigation, or dynamic state transitions are often implemented in monolithic layers.
- Hard-to-Trace Interactions: Debugging UI logic becomes hard when input flows aren't declarative or traceable across boundaries.
MIL introduces a composable, testable, and declarative layer for binding user input to contextual behavior—within and across modular UI boundaries.
Historical Context and Prior Art
1. MVC/MVP: Controller-Centric Input Mapping
Legacy patterns like MVC or MVP coupled interaction tightly with domain controllers. As component boundaries emerged, these input handlers became either too global or too fragmented.
2. Redux and Centralized State
Interaction was routed through central dispatchers or reducers. While powerful, this approach introduced coupling, latency, and state bloating when applied to modular UIs.
3. Event Bus and Pub/Sub Systems
Loose coupling via event buses helped decouple interaction, but lacked traceability and type safety in complex hierarchies.
4. Custom Hooks and Signals
Modern systems like React Hooks or Solid.js signals expose local interaction logic, but lack standard patterns for cross-component interaction at scale.
MIL builds on these ideas—favoring declarative interaction binding, context-aware scoping, and cross-boundary orchestration of interactions.
Architecture Overview
To illustrate how MIL works in real-world applications, consider a user clicking a 'Save' button in a document editor:
- The Intent Emitter captures the click event.
- The Contextual Resolver checks which document is active and whether the user has write permissions.
- The Interaction Handler invokes the logic to persist the document.
- The Feedback Channel displays a toast notification and updates the button state.
MIL is composed of:
- Intent Emitters – Abstracted user actions (click, gesture, voice)
- Contextual Resolvers – Determine what should respond to the intent (via context, scope, or surface)
- Interaction Handlers – Modular, portable logic blocks that execute the behavior
- Feedback Channels – Visual, auditory, or haptic feedback confirming the interaction occurred
+---------------+ +---------------------+ +-------------------+ +------------------+
| User Action | ---> | Intent Emitter | ---> | Interaction Logic | ---> | Feedback Channel |
| (click, etc.) | | (onPress, onVoice) | | (resolve + execute)| | (UI response) |
+---------------+ +---------------------+ +-------------------+ +------------------+
Implementation Examples
Each of these examples aligns with the MIL architecture:
- Intent Emitters are click handlers or custom events.
- Contextual Resolvers are powered by hooks or scoped registrations.
- Interaction Handlers are central logic modules registered with MIL.
- Feedback Channels are controlled through UI state and responses.
React: Modular Click Handling
function SaveButton() {
const onSave = useInteractionHandler('saveAction');
return <button onClick={onSave}>Save</button>;
}
useInteractionHandler
binds an intent ("saveAction") to the behavior registered in MIL.- MIL internally resolves the scope (e.g., user context, device) and invokes the proper handler.
TypeScript: Registering Interaction Logic
registerInteraction('saveAction', async (ctx) => {
await saveDocument(ctx.documentId);
notify('Saved!');
});
- Logic is defined once and mapped to an intent.
- The handler can be swapped, composed, or redirected at runtime.
Web Components
this.addEventListener('press', () => handleInteraction('saveAction'));
- MIL supports decoupled, platform-agnostic interfaces for interaction.
Patterns and Anti-Patterns
📌 Real-World Example:
- Good Pattern: In Adobe Express, each document tool registers its own handlers only when visible. When switching tools, old handlers are unregistered.
- Anti-Pattern: In a large retail app, a single global click handler attempted to manage all actions, causing regressions and race conditions across components.
✅ Patterns
- Scoped Registrations: Handlers bound only in their UI domain or layout scope.
- Intent as ID: Every interaction is identified by a string or token, decoupling it from implementation.
- Cross-Surface Reusability: Same interaction logic on mobile, web, or TV interfaces.
🚫 Anti-Patterns
- Binding to DOM events directly in business logic
- Hardcoded handlers in deeply nested components
- One handler for multiple unrelated behaviors
Real-World Case Studies
Spotify – Unified Playback Controls
Problem: Spotify’s playback logic was fragmented across multiple platforms—web player, mobile apps, car UIs, and smart speakers. Each had slightly different behaviors, causing inconsistencies in seeking, pausing, and state syncing.
Challenge: Developers found it difficult to scale features (like 'jump to chorus') across all platforms while maintaining consistent behavior. Platform-specific hacks increased complexity.
Solution:
- Introduced a MIL pattern where all user interaction was abstracted into high-level intent tokens (e.g.,
playTrack
,skipForward
,seekTo
). - Central interaction handlers were injected contextually based on platform and device type.
- Used a shared analytics layer to trace interaction origin and impact across surfaces.
How They Did It:
- Created an
interactionRegistry
scoped by device type (mobile, embedded, desktop). - Routed all UI actions through an internal
emitIntent('playTrack')
API. - Wrapped platform-specific implementations in a feedback abstraction (e.g., visual play toggle, haptic tap).
Result:
- Reduced interaction bugs by 60% across surfaces
- Improved engineering onboarding time (new controls rolled out in 2× less time)
- Unified playback telemetry enabled smarter UX experiments
📘 Insight: By decoupling UI components from input logic, Spotify dramatically improved cross-platform parity and traceability.
Adobe Express – Action Palette
Problem: Adobe Express needed a way to let users quickly trigger design actions—like aligning objects, changing layout, or applying filters—across various device types.
Challenge: Embedding logic directly into UI controls limited reusability and made accessibility hard to implement consistently.
Solution:
- Introduced a MIL-based command system with scoped handlers.
- Each interaction (e.g.,
alignLeft
,applyFilter
) was treated as a token resolved at runtime. - Floating UI (Command Palette) dispatched these tokens regardless of where the action originated (touch, keyboard, voice).
How They Did It:
- Used React Context to expose interaction registration API per document scope.
- Enabled simulation of intent triggers via automated tests (e.g.,
emitIntent('duplicateElement')
in Cypress). - Composed interaction logic via middleware (e.g., telemetry, feature flag gating).
Result:
- 3× improvement in discoverability of design tools
- Automated interaction test coverage reached 85%
- Team reduced interaction-related bugs in cross-device flows by ~45%
📘 Reference: Adobe Spectrum’s approach to interaction APIs helped inform the MIL structure.
Glossary
Term | Description |
---|---|
Intent Emitter | Abstract source of user input (click, gesture, voice, etc.) |
Interaction Handler | Logic that processes and responds to intent |
Context Resolver | Layer that decides what handler should be triggered |
Feedback Channel | UI, sound, or haptic response confirming user action |
Scope Binding | Restricting interaction logic to a section or view context |
Summary
MIL is the orchestrator of user intent in a composable UI system. It ensures interactions are decoupled, scoped, traceable, and portable—allowing behavior to scale as UIs become more distributed.
✅ Next Steps
- Audit your UI for hardcoded input logic
- Define reusable intent tokens (e.g., 'submitForm', 'togglePlayback')
- Create scoped interaction handlers
- Implement feedback channels for each interaction
- Simulate and test intent flows end-to-end
→ Next: Chapter 8 – The Composable Runtime Shell
Environment logic in core business rules: Placing logic like
if (isEdge)
deep inside shared business workflows leads to tangled dependencies. Instead, move environment selection to the composition layer or infrastructure config, and delegate to environment-specific modules.Scattering
isClient
checks across app: Repeated conditionals across dozens of files cause bugs and make logic brittle. Use environment-aware wrappers or runtime resolvers to abstract away the conditionals.Neglecting hydration mismatches: Server-rendered components that diverge in behavior or layout after hydration break user trust and increase time to interactive. Ensure logic executes identically (or gracefully degrades) between server and client. Testing frameworks like Playwright and Cypress can help validate consistency across runtimes.
How to Test CSEE Workflows
Testing multi-runtime logic requires different layers of validation to ensure consistent behavior across surfaces. Here’s a guide:
🔍 Unit Testing Logic by Runtime
Use flags or mocking tools to simulate environments:
// inside test
Object.defineProperty(global, 'EdgeRuntime', { value: true });
expect(() => executeInContext('edge', fn)).not.toThrow();
🧪 Integration Testing
Use tools like Playwright or Cypress to simulate end-to-end flows across SSR, hydration, and interaction.
📊 Observability
Log when and where each function executes:
console.log(`[CSEE] Running validateOrder on: ${getRuntime()}`);
Use this in combination with APM tools (e.g., Datadog, New Relic) to track latency.
💡 Best Practices
- Test all surfaces in CI: client, edge, server.
- Use shared test suites per logic module.
- Run hydration consistency snapshots.
📘 Tip: CSEE modules should be as pure and environment-agnostic as possible to maximize testability.
Tooling and Developer Experience
Supporting multiple execution contexts introduces complexity—tooling must offset this by offering visibility, simulation, and guardrails.
- Execution Maps: Show which modules are evaluated in client, edge, or server—often visualized via build tools or trace maps.
- Mocked Runtimes: Run client-only or edge-only code locally using
jest-runtime
,vite-ssr
, orwrangler dev
. - Latency Simulators: Profile logic under different network and compute constraints to decide optimal location.
- Deployment Planners: Tools that recommend where logic should live based on runtime availability, performance thresholds, or regulatory constraints.
CSEE Migration Journey
This section provides a concrete path for teams modernizing from traditional execution to the CSEE model.
Step-by-Step
Identify Logic Candidates
Start with logic duplicated across platforms—like validation, pricing, session handling.Encapsulate in Modules
Move that logic into isolated functions with no runtime-specific code. Make sure it's testable.Introduce Runtime Awareness
Wrap logic with an execution context helper (e.g.,executeInContext
) to define where it runs.Deploy to Runtime Surfaces
Use Vercel Edge, Lambda@Edge, SSR routes, or client hydration based on logic needs.Observe & Iterate
Track performance, consistency, and errors. Use observability tooling to tune deployment strategy.
📘 Teams often begin by moving pricing, personalization, or auth checks to the edge, then expand to full workflows.
CI/CD Deployment Patterns
To successfully adopt CSEE in production, you need a CI/CD pipeline that supports bundling logic per environment and deploying it to the appropriate surfaces. Here's how to do that:
📦 Modular Build Outputs
Organize logic modules by target runtime:
src/logic/validateOrder/
├── client.ts // client-only validation logic
├── edge.ts // edge-executable geolocation rules
└── server.ts // server-side payment and compliance checks
🛠 Build Pipeline
- Use conditional entry points or wrappers to target builds for client, server, and edge.
- Tools like esbuild, Vite, or Webpack can create separate runtime bundles.
- CI detects runtime-specific folders and outputs per environment:
# Pseudo build steps
vite build --config client.vite.config.js
vite build --config edge.vite.config.js
vite build --config server.vite.config.js
🚀 Deployment Flow
Step | Tool / Strategy |
---|---|
Runtime Detection | executeInContext() helper |
Edge Deployment | Vercel Edge / Cloudflare Workers |
Server Bundles | SSR Framework (Next.js, Bun) |
Client Distribution | CDN / SPA Loader (React, Vite) |
Observability | Datadog, OpenTelemetry |
By separating logic early and aligning builds with runtime targets, CSEE improves velocity, reliability, and testability across the full delivery lifecycle.
Sample CSEE Stack
Layer | Tooling Example |
---|---|
Runtime Router | TypeScript + feature detection utilities |
Edge Execution | Vercel Edge / Cloudflare Workers |
Server Execution | Node.js + RSC / Bun + API routes |
Client Hydration | React / Web Components |
Testing | Playwright + Jest + CI matrix |
Observability | Datadog, OpenTelemetry, Console Analytics |
Getting Started with CSEE
This checklist provides a structured approach to adopting CSEE incrementally while minimizing risk and maximizing benefit:
Step | Task | Description |
---|---|---|
1 | Define environment boundaries | Identify where your code needs to execute—client, server, edge, or embedded. |
2 | Wrap execution in context helpers | Use utility functions like executeInContext() to abstract away platform checks and conditionals. |
3 | Isolate per-runtime behaviors | Split out hydrate/init/run logic into surface-specific modules to reduce duplication. |
4 | Integrate with runtime tools | Leverage Next.js Middleware, Vercel Edge, Lambda@Edge, or RSC to deploy logic appropriately. |
5 | Monitor and adapt | Use observability tools to track performance, errors, and refine execution placement dynamically. |
Example Walkthrough
Let’s say you want to validate a login request at the edge to reduce round-trips. Here’s how to apply this checklist:
- Define Environment: You want this to run on the edge near the user.
- Wrap with Helper:
executeInContext('edge', () => {
validateLogin(input);
});
- Isolate Logic: Place
validateLogin()
insideedge/validate.ts
and import it conditionally. - Use Runtime Tooling: Deploy via
middleware.ts
in Next.js or Edge Functions on Cloudflare. - Monitor: Log execution latency and fallback counts to determine if re-routing to server is necessary.
Following this approach allows teams to scale logic delivery without brittle isServer
checks or runtime-specific hacks.
Benefits of Adopting CSEE
Performance at the Edge: Reduce latency by running personalization and validation logic close to users.
Real-world usage of CSEE patterns (e.g. in Vercel Edge, Walmart) has shown Time to First Byte (TTFB) improvements of up to 3× in personalized experiences.
Code Reuse: Write logic once and reuse it across client, server, and edge without duplication.
Teams migrating to CSEE reduced duplicated business logic by up to 70%, lowering maintenance overhead across platforms.
Developer Velocity: Modular execution lets teams build independently without deep environment coupling.
One Shopify team accelerated their checkout iterations from weekly to daily cycles by decoupling logic into cross-surface modules.
Reliability and Observability: Better runtime insights and testing make debugging easier and safer.
Centralized telemetry across runtimes led to a 40% reduction in production errors in shared workflows.
Composable Deployments: Logic can be versioned, tested, and shipped per environment or feature slice.
Walmart’s deployment time for logic-layer updates dropped from 5 days to under 12 hours using composable logic blocks.
CSEE in the Wild
Netflix – Thumbnail Optimization at the Edge
Netflix leverages edge functions to determine the most relevant thumbnail or preview trailer based on user locale, preferences, and A/B test conditions—executed before the page reaches the browser.
Shopify – Checkout Extensibility Framework
Shopify enables checkout apps to execute both server-side and at the edge, including logic like field validation, promotional rules, or redirect flows. CSEE-like modularity allows embedded logic to run in the appropriate execution layer per store context.
📘 Learn more: Shopify Checkout Extensibility
Limitations and Caveats
While CSEE brings many benefits, it’s not universally ideal:
- Overhead for Small Projects: Introducing multi-runtime tooling adds complexity that may not pay off early.
- Learning Curve: Teams must understand how to simulate and debug logic across environments.
- Hydration Mismatches: Client/Server divergence is still possible if boundaries aren’t clearly defined.
- Tooling Fragmentation: Edge and runtime platforms evolve quickly, creating integration challenges.
Glossary of Terms
Term | Definition |
---|---|
CSEE | Cross-Surface Execution Engine – orchestrates logic across client, edge, and server. |
Execution Context | The runtime environment where logic executes (client, edge, server). |
Hydration | Attaching client-side interactivity to server-rendered HTML. |
Edge Runtime | Lightweight compute environments close to users (e.g., Cloudflare Workers). |
Runtime Host | The environment actually running logic: browser, server, or edge function. |
SSR (Server-Side Rendering) | Rendering HTML on the server to deliver to clients. |
Init / Hydrate / Cleanup | Lifecycle hooks managing logic setup and teardown across runtimes. |
Composable Deployment | Deploying logic in independently managed, runtime-specific modules. |
Next Steps
- 🔍 Audit duplicated logic across your codebase (e.g., pricing, auth, personalization).
- 🧱 Refactor shared logic into reusable, environment-agnostic modules.
- 🧩 Use
executeInContext()
to target logic for the correct runtime. - 🚀 Deploy to Edge, Server, and Client strategically based on need.
- 📊 Observe and iterate using monitoring tools to evaluate performance and correctness.
Summary
CSEE enables applications to operate as distributed, performance-aware systems—fluidly shifting execution to where it makes the most sense for speed, reliability, or scalability.
It bridges the last architectural layer—delivering truly composable apps that not only render anywhere, but also run anywhere.