The Composable Runtime Shell (CRS)
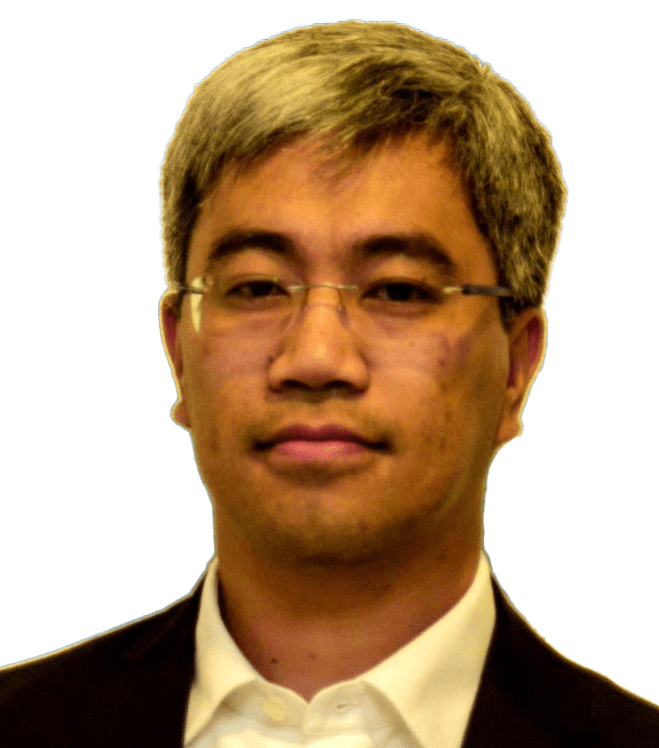
- Published on
Introduction
Picture the experience of launching the Netflix homepage. For each user, it must dynamically stitch together a personalized layout, route to the right module (e.g., kids mode or adult interface), and preload features like 'Continue Watching.' This orchestration is handled by a Composable Runtime Shell (CRS).
In a fully modular frontend system, something must orchestrate the integration of components, handle runtime concerns like loading, routing, authentication, and coordinate between distributed UI fragments. That responsibility belongs to the Composable Runtime Shell (CRS).
CRS is the infrastructure that stitches together modular UI parts into a coherent, user-facing application—at runtime. It is the host, loader, router, state hydrator, and runtime contract enforcer. Think of it as the OS of your composable frontend.
Where MIL manages interaction, CEL handles logic, and DIM structures the view—CRS manages the lifecycle and orchestration of the app itself.
Why We Need CRS
Modern frontend systems are no longer single bundles or even single pages. They're:
- Distributed across micro frontends or independently deployed modules
- Contextual: Personalized per user, region, session, or device
- Performance-sensitive: Need to hydrate fast, defer loads, and optimize transitions
Without a runtime shell:
- Loading becomes chaotic (e.g. waterfall dependencies)
- Routing logic is duplicated across modules
- Global state becomes hard to manage across remounted fragments
- Cross-cutting concerns (auth, telemetry, feature flags) lack a central place
CRS provides the glue layer and contract management system that makes composability practical at runtime.
Responsibilities of CRS
To illustrate, consider how a modular shopping app might behave:
- The shell receives a route to
/shop
- It loads the
shopModule
, passing shared context like user locale and cart ID - When the module fails to load, a fallback UI is shown until retry
Here’s a breakdown of each responsibility:
A well-designed Composable Runtime Shell manages:
- Module Loader: Dynamically loads and initializes UI modules or pages
- Router: Handles navigation events and view transitions across module boundaries
- Shell Context Provider: Offers environment state (user, locale, theme, auth) to all modules
- Lifecycle Manager: Controls mount/unmount cycles and shared subscriptions
- Boundary & Fallback Renderer: Gracefully handles load failures, auth barriers, or slow dependencies
- Integration Contracts: Ensures modules conform to expected APIs, hooks, or conventions
Historical Context and Prior Art
- Single-SPA: Early implementations of runtime shells via route-based mounting of micro frontends
- Module Federation (Webpack): Enables runtime composition via shared code and cross-host imports
- React Router + Context: Paired routing with context providers, but still requires glue code
- Next.js App Router: Server/edge-powered shell routing with slots—but mostly static structure
CRS extends these ideas into a fully dynamic, runtime-configurable shell architecture.
Architecture Diagram
The following diagram illustrates how CRS components work together at runtime:
+--------------------------+
| Navigation Event |
+--------------------------+
|
v
+--------------------------+
| Router |<-------------------------+
| (URL → Module Map) | |
+--------------------------+ |
| |
v |
+--------------------------+ +----------------+ |
| Module Loader +---->+ Remote Module | |
| (dynamic import, retry) | +----------------+ |
+--------------------------+ |
|
v |
+--------------------------+ |
| Shell Context Provider | |
| (auth, locale, theme) | |
+--------------------------+ |
| |
v |
+--------------------------+ |
| Boundary Renderer |<----+ onError hook |
| (suspense, error UI) | |
+--------------------------+ |
| |
v |
+--------------------------+ |
| Mounted UI Module |-------------------------+
| (Rendered component) |
+--------------------------+
**Explanation:**
- **Router**: Maps incoming routes to lazy-loaded modules.
- **Module Loader**: Dynamically imports only the module needed for the current route.
- **Context Provider**: Supplies app-wide data like authentication, theme, locale.
- **Boundary Renderer**: Ensures loading and error boundaries exist during slow loads or failures.
- **Mounted UI Module**: Final composed module mounted into the app view.
8 });
React Shell Host
Explanation:
- Line 2: Retrieves the dynamically resolved component and shared context.
- Line 4: Wraps the rendered module in global context providers (theme, locale, auth).
1 function AppShell() {
2 const { Component, context } = useShell();
3 return (
4 <ShellProvider value={context}>
5 <Component />
6 </ShellProvider>
7 );
8 }
Web Component Registration
Explanation:
- Dynamically imports a UI module and defines it as a Web Component.
- Allows modular, runtime extensibility of UI without bundling everything upfront.
1 shell.register('profile-card', async () => {
2 const module = await import('./profile-card.js');
3 customElements.define('profile-card', module.default);
4 });
Patterns and Anti-Patterns
📌 Example:
- ✅ Netflix’s CRS assigns preload priorities to modules like 'HomePage' and 'KidsUI' using capability flags.
- 🚫 A large eCommerce app experienced runtime bugs due to modules mutating global app state rather than consuming context via the shell.
✅ Patterns
- Shell owns routing, context, and error boundaries
- Modules declare what they need (context, slots, dependencies)
- Shell manages loading strategy (e.g. preload, lazy, retry)
🚫 Anti-Patterns
- Modules modifying shell context directly
- Shell exposing low-level globals
- Deep links depending on fragile module internals
Real-World Case Studies
Netflix – Entrypoint Shell
Problem: Netflix supports a wide variety of interfaces—from smart TVs to mobile apps—each needing fast startup and customized experiences based on profiles, tests, and regions.
Challenge: Without a runtime shell, personalization logic, module bootstrapping, and routing logic were tightly coupled to the view layer. This led to hard-to-maintain logic paths, duplication, and bloated bundles.
Solution:
- Built a CRS that dynamically loads modules using capability flags (e.g.,
requiresAuth
,supportsKidsMode
). - The shell inspects user/device profile and preloads the appropriate UI layer.
- Centralized context injection (e.g., experiments, themes, user permissions).
How They Did It:
- Defined a runtime registry of modules with metadata.
- Used predictive preloading for common user flows (e.g., Home → Continue Watching → Player).
- Enforced shell contract linting to catch misconfigured modules before runtime.
Result:
- ⏱ Cold start time improved by ~40%
- 🔒 Module consistency across devices enforced via shell APIs
- 📉 Reduced runtime errors with shell-level enforcement
Shopify Hydrogen – Server Runtime Shell
Problem: Shopify needed a seamless way to deliver dynamic, server-rendered commerce experiences while preserving fast, personalized client transitions.
Challenge: The system had to handle real-time context (cart, user), dynamic data fetching, and cross-boundary hydration without causing flickers, mismatches, or failures.
Solution:
- Built the Hydrogen shell to orchestrate server-to-client transitions and hydrate only essential parts.
- Every module consumed shell-provided context for cart, auth, and localization.
- Fallback boundaries wrapped all lazy-loaded components.
How They Did It:
- Wrapped view trees in
ShopifyProvider
, which unified server and client logic. - Defined shell integration contracts to standardize module hydration behavior.
- Created server-aware preloads and streaming fallbacks (e.g., placeholder skeletons).
Result:
- 💡 Fully dynamic personalization without hydration bugs
- 🚀 Smooth module loading across edge and client
- 🧪 Test coverage improved with shell-level simulation hooks
Migration Workflow
To successfully migrate toward a composable runtime shell, follow this step-by-step process:
- Audit Existing Glue Code: List where loading, routing, and global context are imperatively scattered.
- Wrap Concerns into Shell Abstractions: Build a wrapper component (e.g.,
<AppShell>
) that owns routing and context provisioning. - Refactor Modules: Update components to consume context (auth, theme, feature flags) from shell-provided APIs.
- Centralize Routing: Move navigation and route maps to the shell config.
- Add Fallback Boundaries: Ensure that all module loads are wrapped in suspense or error boundaries.
- Run Partial Boot Tests: Simulate runtime with only half of modules loaded to verify graceful degradation.
Benefits of CRS
Benefit | Description |
---|---|
Faster Cold Starts | Optimize what modules load and when—prioritize what the user sees first |
Less Duplication | Centralized routing, context, and loader logic removes boilerplate |
Improved Testability | Isolate module behavior and simulate shell states with confidence |
Consistent UX | Reliable fallback and loading strategies keep experience smooth |
Runtime Contracts | Prevents integration issues by enforcing shape and context expectations |
Glossary
Term | Description |
---|---|
Composable Runtime Shell | Host system that coordinates module loading, routing, and app orchestration |
Context Bridge | Provider that makes global state available to each module |
Boundary Renderer | UI fallback shown when modules fail or take too long to load |
Shell Contract | API or lifecycle shape that modules must implement to plug into the shell |
CRS Starter Checklist
- ✅ Define shell context contract (user, locale, auth)
- ✅ Map modules to dynamic routes
- ✅ Add error boundaries and fallback renderers
- ✅ Build and test shell context providers
- ✅ Simulate partial system boot to validate resilience
Summary
CRS enables composable frontends to work as a unified product. It provides the runtime environment, coordination logic, and platform-level boundaries needed for dynamic, distributed UIs.
It’s not just a router or loader—it’s the foundation layer for making composable systems feel cohesive to the end user.