Dynamic Interface Mesh (DIM)
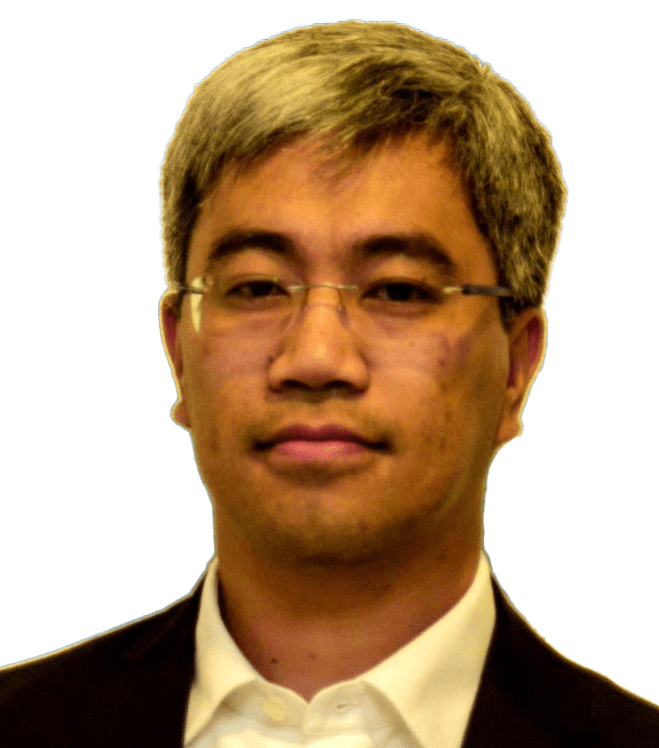
- Published on
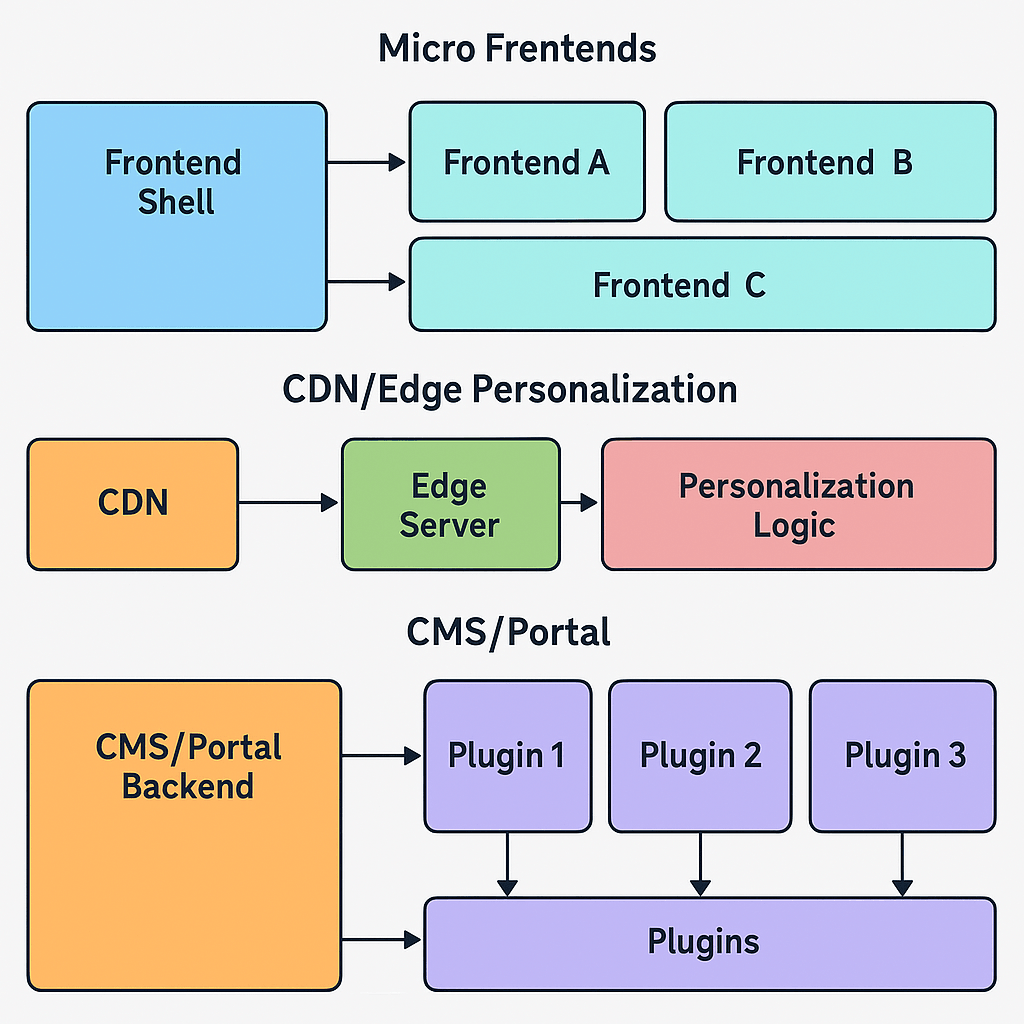
A Flexible, Reactive Architecture for Modern UIs
Introduction to DIM
The Dynamic Interface Mesh (DIM) is an innovative architectural approach that allows for the seamless integration of dynamic, highly-interactive user interfaces (UIs) with backend services and data sources. DIM focuses on creating flexible, data-driven interfaces that adapt to changing conditions in real-time. It is designed to allow for modular, reusable components that dynamically update and interact with different systems.
DIM aims to simplify the development of complex UIs by creating a mesh of dynamic interfaces that can be dynamically composed and reconfigured. This approach allows for a more reactive, adaptable UI architecture that can evolve with the needs of both users and developers.
Why DIM?
With traditional frontend architectures, UI components are often tightly coupled with business logic and static views. As modern applications grow in complexity and need to support a wide range of devices, it's essential to rethink how UIs are structured. DIM addresses this challenge by allowing UIs to be composed dynamically, based on user interaction, state changes, or external system events. This creates a more reactive and modular approach to UI development.
Core Principles of DIM
Dynamic Composition: DIM supports the dynamic composition of UI components. Rather than statically defining all the components in advance, developers can define dynamic compositions that adapt to user input or external data.
Reactive and Real-Time Updates: DIM leverages reactive programming principles to update UIs in real-time, ensuring that any changes in data or user interaction are immediately reflected in the interface.
Modular and Reusable Components: The modularity of DIM allows developers to create reusable, independent UI components that can be composed together in various configurations. These components can be combined and recombined dynamically based on the current state of the application.
Mesh of Interfacing Components: DIM envisions a mesh of components interacting with each other via well-defined interfaces. These components are loosely coupled, allowing them to interact with one another dynamically without direct dependencies.
How DIM Works: Architecture Breakdown
DIM is based on an architecture that decouples the UI, backend logic, and external services into independent, loosely-coupled components. These components are then dynamically composed to form a complete UI based on the current state and needs of the application.
1. Dynamic Components
DIM relies on dynamic components that are independent of each other but can be composed together to create a rich, interactive UI. These components can change their behavior, content, and layout based on the application's state.
Example: Dynamic Button Component in React
// DynamicButton.js
import React from 'react';
const DynamicButton = ({ label, onClick, style }) => {
return (
<button onClick={onClick} style={style}>
{label}
</button>
);
};
export default DynamicButton;
The DynamicButton component is highly reusable and can be used in different parts of the application. Its behavior (e.g., the onClick
handler) and style can be customized dynamically based on external conditions.
2. Mesh of Interfaces
The mesh of interfaces connects various components together through well-defined contracts or interfaces. Each component communicates with others through these interfaces, enabling a modular and extensible system where components can interact with each other dynamically.
Example: Interface for Fetching Data
// IDataFetcher.js
class IDataFetcher {
fetchData() {
throw new Error("Method not implemented");
}
}
export default IDataFetcher;
Here, the IDataFetcher contract defines the interface for fetching data, ensuring that any component that implements this interface can be used by other components without worrying about how data is fetched or processed.
3. Adapters
Adapters are responsible for connecting the dynamic components to the backend services or external APIs. These adapters provide the necessary implementation of the interfaces and convert the data into a format that the UI can consume.
Example: Adapter for Fetching User Data
// UserDataAdapter.js
import IDataFetcher from './IDataFetcher';
class UserDataAdapter extends IDataFetcher {
constructor(apiUrl) {
super();
this.apiUrl = apiUrl;
}
async fetchData() {
const response = await fetch(this.apiUrl);
const data = await response.json();
return data;
}
}
export default UserDataAdapter;
In this example, the UserDataAdapter is responsible for fetching data from an API. It implements the IDataFetcher contract and converts the response into a usable format for the UI.
4. Dynamic Compositions in React
In DIM, the UI is composed dynamically by combining different components based on the application's state. In React, this is achieved by rendering components conditionally or passing dynamic props to control their behavior.
Example: Dynamic Composition of Components
// DynamicInterface.js
import React, { useState, useEffect } from 'react';
import DynamicButton from './DynamicButton';
import UserDataAdapter from './UserDataAdapter';
const DynamicInterface = () => {
const [userData, setUserData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
const adapter = new UserDataAdapter('https://api.example.com/user');
try {
const data = await adapter.fetchData();
setUserData(data);
} catch (err) {
setError('Failed to load user data');
} finally {
setLoading(false);
}
};
fetchData();
}, []);
const handleClick = () => {
alert('Button clicked');
};
return (
<div>
{loading && <p>Loading...</p>}
{error && <p>{error}</p>}
{userData && (
<div>
<h1>{userData.name}</h1>
<DynamicButton label="Click Me" onClick={handleClick} style={{ color: 'blue' }} />
</div>
)}
</div>
);
};
export default DynamicInterface;
In the DynamicInterface component, we dynamically compose the UI by fetching user data using an adapter and rendering the DynamicButton component based on the state. The button’s behavior can be customized dynamically, such as changing the label, style, or click handler.
Benefits of DIM
Modularity: DIM encourages the creation of independent, reusable components that can be easily composed together. This reduces duplication of logic and enhances maintainability.
Reactivity: DIM leverages reactive programming principles to update the UI automatically in response to changes in state or external data, ensuring that users always see the most up-to-date information.
Flexible UI Composition: With DIM, UI components can be composed dynamically based on user interactions or state changes, making the UI more flexible and responsive to evolving requirements.
Scalability: DIM allows for scalable application architectures, where new components can be added without disrupting the existing system. This makes it easier to scale both the frontend and backend independently.
Applications of DIM
1. Real-Time Applications
DIM is well-suited for applications that require real-time updates, such as dashboards, live feeds, and messaging systems. The reactive nature of DIM ensures that UI components are always in sync with the underlying data.
2. Data-Driven Interfaces
DIM is ideal for applications that are data-driven and need to display dynamic content based on real-time data from external sources. Examples include data visualization tools, e-commerce sites, and content management systems (CMS).
Conclusion
The Dynamic Interface Mesh (DIM) provides a flexible, reactive architecture for building modern user interfaces. By enabling the dynamic composition of modular components, DIM allows for the creation of adaptive and real-time UIs. Whether you're building dashboards, e-commerce platforms, or data-driven applications, DIM offers a robust solution that simplifies the development and maintenance of complex, dynamic interfaces.
With DIM, you can focus on creating high-quality, reusable components while ensuring that your applications remain responsive to user interactions and external data.