Cross-Surface Execution Engine (CSEE)
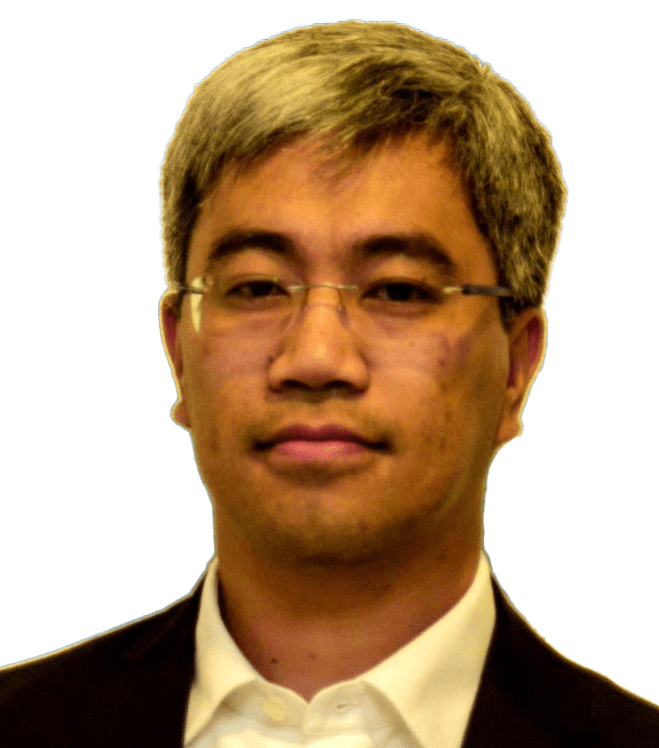
- Published on
Execute Once, Run Anywhere Across UI Surfaces
Introduction to CSEE
The Cross-Surface Execution Engine (CSEE) is a foundational runtime concept that allows application logic to execute uniformly across varied UI surfaces, including web, mobile, desktop, embedded systems, and even non-visual interfaces (voice, automation). It acts as the execution backbone that supports context-aware runtime orchestration, enabling a truly composable and reactive system across environments.
CSEE ensures your code is agnostic of where it's running, while still having access to surface-specific capabilities through pluggable adapters. It's designed for multi-platform frontends, where the same business logic and workflows are shared, yet tailored for the execution context.
Why CSEE?
Modern applications are no longer limited to a single platform. They must be:
- Responsive across screen sizes and devices
- Adaptive to server/client rendering conditions
- Reactive to interaction modalities (click, voice, sensors, API)
- Consistent in business logic execution
But each surface brings its own challenges—rendering model, lifecycle constraints, capabilities, latency, and interaction paradigms.
CSEE resolves these by decoupling execution logic from rendering surfaces, enabling developers to build once and execute adaptively across any context.
Key Goals of CSEE
Context-Aware Execution
Execution adapts dynamically to runtime capabilities (e.g., server-side rendering, client hydration, native OS constraints).Environment-Driven Lifecycle
CSEE interprets and reacts to lifecycle signals (e.g., mount, visibility, resume) from the hosting surface.Composable Execution Contracts
Business logic and workflows are composed into execution graphs that can be reused across different surfaces.Decoupled from Rendering
CSEE does not assume any rendering pipeline—it simply provides state, commands, and feedback.Unified Control Layer
Acts as the orchestration engine beneath Adaptive Presentation Core (APC) or Universal Interaction Framework (UIF).
CSEE Architecture Overview
CSEE is built around three key constructs:
- Execution Units: Small runtime-agnostic behaviors (e.g., auth checks, data flows, side effects).
- Context Adapters: Plug-in adapters that expose runtime surface features (e.g., cookies in SSR, viewport on client).
- Lifecycle Orchestrator: Coordinates when and how execution occurs based on lifecycle signals from the host environment.
Code Example: Cross-Surface User Login Flow
Let’s walk through an example login flow executed through CSEE, using React as one rendering context.
1. Define an Execution Unit
// csee/executions/loginFlow.js
export const loginFlow = async ({ getContext, execute, emit }) => {
const { username, password } = getContext('form');
emit('auth:started');
try {
const token = await execute('authService.login', { username, password });
emit('auth:success', token);
} catch (err) {
emit('auth:failure', err);
}
};
This is a cross-surface execution unit. It could run:
- In the browser (on button press)
- On the server (SSR or edge function)
- On a mobile app (native login screen)
2. React Hook Adapter
// csee/adapters/useCSEE.js
import { useState, useCallback } from 'react';
export const useCSEE = (unit, context = {}) => {
const [state, setState] = useState({ status: 'idle' });
const run = useCallback(async () => {
setState({ status: 'running' });
const getContext = (key) => context[key];
const execute = async (service, payload) => {
if (service === 'authService.login') {
return fetch('/api/login', {
method: 'POST',
body: JSON.stringify(payload),
headers: { 'Content-Type': 'application/json' }
}).then(res => res.json());
}
};
const emit = (event, payload) => {
setState({ status: event, data: payload });
};
await unit({ getContext, execute, emit });
}, [context]);
return [state, run];
};
3. Rendered in React Component
// LoginForm.js
import React, { useState } from 'react';
import { loginFlow } from './executions/loginFlow';
import { useCSEE } from './adapters/useCSEE';
const LoginForm = () => {
const [form, setForm] = useState({ username: '', password: '' });
const [state, run] = useCSEE(loginFlow, { form });
return (
<form onSubmit={(e) => { e.preventDefault(); run(); }}>
<input
type="text"
placeholder="Username"
value={form.username}
onChange={(e) => setForm({ ...form, username: e.target.value })}
/>
<input
type="password"
placeholder="Password"
value={form.password}
onChange={(e) => setForm({ ...form, password: e.target.value })}
/>
<button type="submit">Login</button>
{state.status === 'auth:failure' && <p>Login failed!</p>}
</form>
);
};
Surface Adapters
CSEE can plug into multiple runtime adapters:
- Client Adapter: Runs on the browser, hydrated with context like localStorage, URL, viewport, etc.
- Server Adapter: Runs during SSR or at the edge.
- Native Adapter: Executes in mobile/desktop environments.
- Headless Adapter: Executes logic for headless rendering (PDF, screenshot, static generation).
Each adapter plugs into lifecycle events (onMount
, onVisible
, onSubmit
) and provides context to the engine.
Use Cases for CSEE
- Shared Workflows: Reuse the same login or payment flow across mobile, web, and embedded.
- Composable Automation: Trigger workflows from user events or external systems (webhooks, schedulers).
- Platform-Agnostic Business Logic: Keep domain logic out of rendering concerns.
- Performance Tuning: Execute heavier logic on the server, lighter logic on the client—without changing the source code.
Benefits of CSEE
✅ Unified Runtime Behavior
✅ Improved Developer Experience
✅ Clear Separation of Concerns
✅ Consistent User Flows
✅ Surface-Specific Adaptability
✅ Strong Debuggability and Observability
✅ Better Reusability Across Teams
Conclusion
The Cross-Surface Execution Engine (CSEE) unlocks the power of consistent, composable, and context-aware application logic. It frees developers from duplicating logic across platforms while enhancing agility, performance, and code quality.
When paired with the Composable Execution Layer (CEL), Adaptive Presentation Core (APC), and Universal Interaction Framework (UIF), CSEE forms the runtime heart of a truly platform-independent frontend system.